STEP2: My first angular bean
create a new packageexamples.angularbeans.beans
and a new class: HelloBean
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | package examples.angularbeans; import angularBeans.api.http.Get; import angularBeans.api.AngularBean; import angularBeans.api.NGModel; import angularBeans.api.NGReturn; import angularBeans.api.NGSubmit; @AngularBean public class HelloBean { private String message; private String name = "insert your name here"; @Get @NGSubmit(backEndModels = "name") @NGReturn(updates = "message") public void sayHello() { message = "Hello " + name + "from angularBeans !"; } @NGModel public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } @NGModel public String getName() { return name; } public void setName(String name) { this.name = name; } } |
webapp/index.html
file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | <!DOCTYPE html> <html ng-app="myModule"> <head> <meta charset="ISO-8859-1"> <title>example1</title> <script type="text/javascript" src="bower_components/angular/angular.min.js"></script> <script type="text/javascript" src="angular-beans.js"></script> <script type="text/javascript"> angular.module("myModule", [ "angularBeans" ]).controller("FirstCtrl", function($scope, helloBean) { $scope.helloBean = helloBean; }); </script> </head> <body ng-controller="FirstCtrl"> <input type="text" ng-model="helloBean.name"> <label>Server Response:</label> <h2>{{helloBean.message}}</h2> <button ng-click="helloBean.sayHello()">say hello</button> </body> </html> |
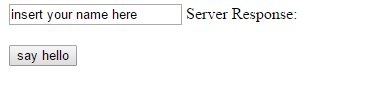
what's happened?
let'start by explaing the Java CDI part: the@AngularBean
annotation decalre a CDI bean
acceccible remotely by a javascript proxy.
by default, an Angular Bean is a RequestSoped bean unless we annotate them as NGSessionScoped or ApplicationScoped (we will talk about scopes later..).
by remotely callabable we mean all the public methods declared within the class (excluding getters & setters). so what is the
@GET
annotation?It's simply an explicite way to tell angularBeans that the annotated method will be remotely called by a HTTP GET method Request. This is the default behavioure so you can simply omit that if you want a GET method, but also it can be @POST,@DELETE,@PUT http request, you have just to add the specified annotation to the bean method,
But anguarBeans came with a new annotaion: @RealTime, we will discuss this case later. observe now the
@NGModel
annotated getters, (and it should be on top of getters), those are properties that define equivalent properties in the javascript proxy side.
Now look at the field name, it's an initialized String with the value "insert your name here", the js proxy "helloBean" will have the same property "name" and this propertie will be initialized by this value (as shown in the browser snapshot);
We turn back to ower sayHello() method, there are 2 others annotations to describe :
@NGSubmit:
this annotation and its attribute backEndModels tooks an array (or single String) that list the @NGModel annotated properties to synchronize when the request is triggered. that give you a fine controle of data that will be send from browsers to the server.(if you omit this annotation none of all the @NGModel annotated properties will be synchronized).
In the other hands if you use @NGSubmit(backEndModels="*") all the backend models will be updated with the javascript bean proxy state before the execution of the method.
All that is applicable for the @NGReturn annotation but in the other way (server to client) and this synchronization will happen after the method execution.
HTML/angularJS code:
Most of the code is a regular angularjs application code except few points:The
<script type="text/javascript" src="angular-beans.js"></script>
call a server generated javascript, that include an angularJS module called "angularBeans".
This module will be used as dependency on your angularJS application module(s) at line 11.
After that (at line 12) we inject a generated angularJs service in the controller function: "helloBean". (pay attention at the lowerCase first caractere of the service name).
At line 13 we affect the helloBean object reference to a property of the $scope, that allow us to use ower angular-bean inside the cotroller bound scope.
I think the rest of the code is easy to undrestand now :)
next step:
<STEP3: angularBean and RPC style>
Support or Contact
Having trouble with Pages? Check out the documentation at https://help.github.com/pages or contact support@github.com and we’ll help you sort it out.