STEP3: angularBean RPC style
we will now make changes on the previous HelloBean class: by removing the "message" property ower class will look like:1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | package examples.angularbeans; import angularBeans.api.http.Get; import angularBeans.api.AngularBean; import angularBeans.api.NGModel; import angularBeans.api.NGSubmit; @AngularBean public class HelloBean { private String name = "insert your name here"; @Get @NGSubmit(backEndModels = "name") public String sayHello() { return "Hello " + name + " from angularBeans !"; } @NGModel public String getName() { return name; } public void setName(String name) { this.name = name; } } |
and here is the changes on the "index.html" page:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | <!DOCTYPE html> <html data-ng-app="myModule"> <head> <meta charset="ISO-8859-1"> <title>example1</title> <script type="text/javascript" src="bower_components/angular/angular.min.js"></script> <script type="text/javascript" src="angular-beans.js"></script> <script type="text/javascript"> angular.module("myModule", [ "angularBeans" ]).controller("FirstCtrl", function($scope, helloBean) { $scope.name = helloBean.name; $scope.sayHello = function(name) { helloBean.name = name; helloBean.sayHello().then(function(data) { $scope.message = data; }); } }); </script> </head> <body data-ng-controller="FirstCtrl"> <input type="text" data-ng-model="name"> <label>Server Response:</label> <h2>{{message}}</h2> <button data-ng-click="sayHello(name)">say hello</button> </body> </html> |
explanation:
With those changes we deal more with the angularJs controller "FirstCtrl", so in the mustache expressions we use properties from the scope, and we create a scoped function called sayHello(name){...}, this way we create an abstraction of ower angularBean for the "view" part of angularJs. but we will have more (price-to-pay) js code: initialising the $scope "name" model, and dealing with the return of ower HTTP call, but look, now the java sayHello() method of the bean is no more a no return (void) method, it return a String, that tell angularBean that the return value will be the promise data. and no more need to the NGReturn annotation.Angular bean have another more RPC style for remotes calls :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | package examples.angularbeans; import angularBeans.api.http.Get; import angularBeans.api.AngularBean; import angularBeans.api.NGModel; @AngularBean public class HelloBean { private String name = "insert your name here"; @Get public String sayHello(String name) { this.name = name; return "Hello " + name + " from angularBeans !"; } @NGModel public String getName() { return name; } public void setName(String name) { this.name = name; } } |
that will give us the next html:
1 2 3 4 5 6 7 8 9 10 11 12 | <script type="text/javascript"> angular.module("myModule", [ "angularBeans" ]).controller("FirstCtrl", function($scope, helloBean) { $scope.name = helloBean.name; $scope.sayHello = function(name) { // helloBean.name = name; //no needs to that now helloBean.sayHello(name).then(function(data) { $scope.message = data; }); } }); </script> |
cool? and an angularBean method can took any java base type arguments,an array, any nomber of arguments, event java var args (as an array on the javaScript side). they also support overloading (but you must change the arguments number). also you can pass as parametre a custom object. let's change the "sayHello" Method on both sides:
add the following java class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | package examples.angularbeans; public class Language { private String value; public String getValue() { return value; } public void setValue(String value) { this.value = value; } } |
now update the sayHello() method:
1 2 3 4 5 6 | @Get public String sayHello(String name, Language language) { this.name = name; return "Hello " + name + " from angularBeans, your language is " + language.getValue(); } |
and:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <script type="text/javascript"> angular.module("myModule", [ "angularBeans" ]).controller("FirstCtrl", function($scope, helloBean) { $scope.name = helloBean.name; $scope.sayHello = function(name) { var language={value:"French"}; // helloBean.name = name; //no needs to that now helloBean.sayHello(name,language).then(function(data) { $scope.message = data; }); } }); </script> |
And you will get:
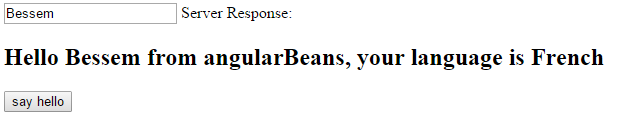
next step:
<STEP4: binding>
Support or Contact
Having trouble with Pages? Check out the documentation at https://help.github.com/pages or contact support@github.com and we’ll help you sort it out.